Prism(五) 命令
源码地址 - WineMonk/PrismStudy: Prism框架学习 (github.com)
委托命令
MainWindow.xaml
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15
| <Window x:Class="UsingDelegateCommands.Views.MainWindow" xmlns="http://schemas.microsoft.com/winfx/2006/xaml/presentation" xmlns:x="http://schemas.microsoft.com/winfx/2006/xaml" xmlns:prism="http://prismlibrary.com/" prism:ViewModelLocator.AutoWireViewModel="True" Title="Using DelegateCommand" Width="350" Height="275"> <StackPanel HorizontalAlignment="Center" VerticalAlignment="Center"> <CheckBox IsChecked="{Binding IsEnabled}" Content="Can Execute Command" Margin="10"/> <Button Command="{Binding ExecuteDelegateCommand}" Content="DelegateCommand" Margin="10"/> <Button Command="{Binding DelegateCommandObservesProperty}" Content="DelegateCommand ObservesProperty" Margin="10"/> <Button Command="{Binding DelegateCommandObservesCanExecute}" Content="DelegateCommand ObservesCanExecute" Margin="10"/> <Button Command="{Binding ExecuteGenericDelegateCommand}" CommandParameter="Passed Parameter" Content="DelegateCommand Generic" Margin="10"/> <TextBlock Text="{Binding UpdateText}" Margin="10" FontSize="22"/> </StackPanel> </Window>
|
MainWindowViewModel.cs
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 48 49 50 51 52 53 54 55 56
| public class MainWindowViewModel : BindableBase { private bool _isEnabled; public bool IsEnabled { get { return _isEnabled; } set { SetProperty(ref _isEnabled, value); ExecuteDelegateCommand.RaiseCanExecuteChanged(); } }
private string _updateText; public string UpdateText { get { return _updateText; } set { SetProperty(ref _updateText, value); } }
public DelegateCommand ExecuteDelegateCommand { get; private set; }
public DelegateCommand<string> ExecuteGenericDelegateCommand { get; private set; }
public DelegateCommand DelegateCommandObservesProperty { get; private set; }
public DelegateCommand DelegateCommandObservesCanExecute { get; private set; }
public MainWindowViewModel() { ExecuteDelegateCommand = new DelegateCommand(Execute, CanExecute);
DelegateCommandObservesProperty = new DelegateCommand(Execute, CanExecute).ObservesProperty(() => IsEnabled);
DelegateCommandObservesCanExecute = new DelegateCommand(Execute).ObservesCanExecute(() => IsEnabled);
ExecuteGenericDelegateCommand = new DelegateCommand<string>(ExecuteGeneric).ObservesCanExecute(() => IsEnabled); }
private void Execute() { UpdateText = $"Updated: {DateTime.Now}"; }
private void ExecuteGeneric(string parameter) { UpdateText = parameter; }
private bool CanExecute() { return IsEnabled; } }
|
复合命令
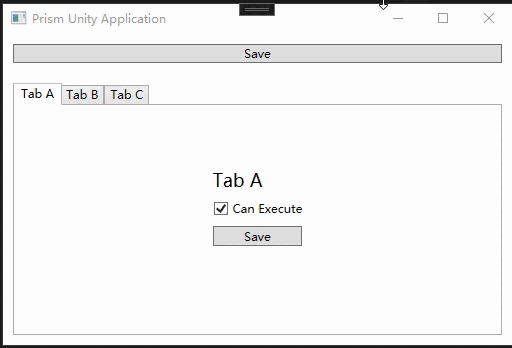
TabView模块
TabView.xaml
1 2 3 4 5 6 7 8 9 10 11 12
| <UserControl x:Class="ModuleA.Views.TabView" xmlns="http://schemas.microsoft.com/winfx/2006/xaml/presentation" xmlns:x="http://schemas.microsoft.com/winfx/2006/xaml" xmlns:prism="http://prismlibrary.com/" prism:ViewModelLocator.AutoWireViewModel="True"> <StackPanel VerticalAlignment="Center" HorizontalAlignment="Center"> <TextBlock Text="{Binding Title}" FontSize="18" Margin="5"/> <CheckBox IsChecked="{Binding CanUpdate}" Content="Can Execute" Margin="5"/> <Button Command="{Binding UpdateCommand}" Content="Save" Margin="5"/> <TextBlock Text="{Binding UpdateText}" Margin="5"/> </StackPanel> </UserControl>
|
TabViewViewModel.cs
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41
| public class TabViewModel : BindableBase { IApplicationCommands _applicationCommands;
private string _title; public string Title { get { return _title; } set { SetProperty(ref _title, value); } }
private bool _canUpdate = true; public bool CanUpdate { get { return _canUpdate; } set { SetProperty(ref _canUpdate, value); } }
private string _updatedText; public string UpdateText { get { return _updatedText; } set { SetProperty(ref _updatedText, value); } }
public DelegateCommand UpdateCommand { get; private set; }
public TabViewModel(IApplicationCommands applicationCommands) { _applicationCommands = applicationCommands;
UpdateCommand = new DelegateCommand(Update).ObservesCanExecute(() => CanUpdate);
_applicationCommands.SaveCommand.RegisterCommand(UpdateCommand); }
private void Update() { UpdateText = $"Updated: {DateTime.Now}"; } }
|
公共模块
IApplicationCommands.cs
1 2 3 4 5 6 7 8 9 10 11 12 13
| public interface IApplicationCommands { CompositeCommand MyCompositeCommand { get; } }
public class ApplicationCommands : IApplicationCommands { private CompositeCommand _saveCommand = new CompositeCommand(); public CompositeCommand MyCompositeCommand { get { return _saveCommand; } } }
|
主程序
MainWindow.xaml
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25
| <Window x:Class="UsingCompositeCommands.Views.MainWindow" xmlns="http://schemas.microsoft.com/winfx/2006/xaml/presentation" xmlns:x="http://schemas.microsoft.com/winfx/2006/xaml" xmlns:prism="http://prismlibrary.com/" prism:ViewModelLocator.AutoWireViewModel="True" Title="{Binding Title}" Height="350" Width="525">
<Window.Resources> <Style TargetType="TabItem"> <Setter Property="Header" Value="{Binding DataContext.Title}" /> </Style> </Window.Resources> <Grid> <Grid.RowDefinitions> <RowDefinition Height="Auto"/> <RowDefinition Height="*" /> </Grid.RowDefinitions>
<Button Content="Save" Margin="10" Command="{Binding ApplicationCommands.SaveCommand}"/>
<TabControl Grid.Row="1" Margin="10" prism:RegionManager.RegionName="ContentRegion" /> </Grid> </Window>
|
App.xaml.cs
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17
| public partial class App : PrismApplication { protected override Window CreateShell() { return Container.Resolve<MainWindow>(); }
protected override void ConfigureModuleCatalog(IModuleCatalog moduleCatalog) { moduleCatalog.AddModule<ModuleA.ModuleAModule>(); }
protected override void RegisterTypes(IContainerRegistry containerRegistry) { containerRegistry.RegisterSingleton<IApplicationCommands, ApplicationCommands>(); } }
|
复合命令扩展:指向当前活动视图的命令
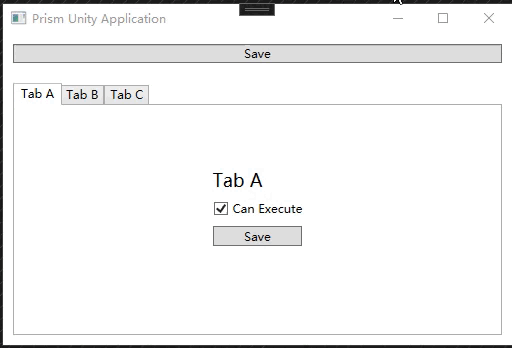
TabViewModel.cs
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 48 49 50 51 52 53 54 55 56 57 58 59 60 61
| public class TabViewModel : BindableBase, IActiveAware { IApplicationCommands _applicationCommands;
private string _title; public string Title { get { return _title; } set { SetProperty(ref _title, value); } }
private bool _canUpdate = true; public bool CanUpdate { get { return _canUpdate; } set { SetProperty(ref _canUpdate, value); } }
private string _updatedText; public string UpdateText { get { return _updatedText; } set { SetProperty(ref _updatedText, value); } }
public DelegateCommand UpdateCommand { get; private set; }
public TabViewModel(IApplicationCommands applicationCommands) { _applicationCommands = applicationCommands;
UpdateCommand = new DelegateCommand(Update).ObservesCanExecute(() => CanUpdate);
_applicationCommands.SaveCommand.RegisterCommand(UpdateCommand); }
private void Update() { UpdateText = $"Updated: {DateTime.Now}"; } bool _isActive; public bool IsActive { get { return _isActive; } set { _isActive = value; OnIsActiveChanged(); } } private void OnIsActiveChanged() { UpdateCommand.IsActive = IsActive;
IsActiveChanged?.Invoke(this, new EventArgs()); } public event EventHandler IsActiveChanged; }
|